A significant number of refund scammers rely on remotely accessing the victim's computer, having them log into their online banking, and then surreptitiously editing the content of the displayed web page to make it look like they have a higher balance (due to their erroneous "refund") and force them to transfer that money, or pay it back by other means (e.g. Google Play Cards or similar)
Indeed, there's been a move by some banks (notably most recently Virgin Money) to move to an app-based banking solution which entirely replaces the web browser one. They cite increased security as a reason for this change, and I expect it's at least partially due to the fact that mobile banking apps can't be attacked in this manner.
However, there's a (simple!) way banks could be helping to prevent this but - as far as I can see - it's not been implemented by any (or many!) of them for reasons I can't understand.
The attack
There's far better explanations out there of how this attack works, and good video demonstrations showing it in action;
In it's simplest form, an attacker opens the Developer Tools of the web browser and this allows them to edit any element on the web page (e.g. changing a balance from $134.00 to $10,134.00 trivially), these changes will disappear with the next page load as they don't 'attack' the bank system in any way, the change is purely to what's displayed on the browser, just like editing a text document.
Some use other techniques, but they all rely on the same thing, changing the web page content as it's displayed in order to trick a victim into believing they have accidentally received a huge sum of money and that they need to give it back to the scammer (and of course the scammer can't let them just pay it back via their online banking)
Usually this is accompanied by a pathetic attempt at social engineering, a sob story about how they'll lose their job or something like that if they don't get the money back (personally, I'm the heartless type, if someone was really that incompetent to send someone $10,000s they probably shouldn't be working in that role anyway!)
Detecting Changes
So, if we can detect these changes, we can fight back against the scammer.
Turns out there's a really simple method for doing this built into almost every modern browser; MutationObserver()
The MutationObserver interface allows us to 'watch' the content of a webpage for changes. Now, most modern web interfaces will make dynamic changes to a web page legitimately but it gives us a way to determine if someone is changing the web page.
Demonstration
If you're viewing this on a desktop browser, right-click the text of the bank balance below, select Inspect Element, and change the value of this bank account. (this is effectively what the scammers do)
Current Account 10-22-30 12345678 |
Balance $1,304.30 |
You'll see an alert that the value has been tampered with, and the changed value will be reset, rendering this type of attack ineffective.
If you're not viewing on a browser that supports Inspect, then a short animation of how this demo behaves in Firefox is included below;
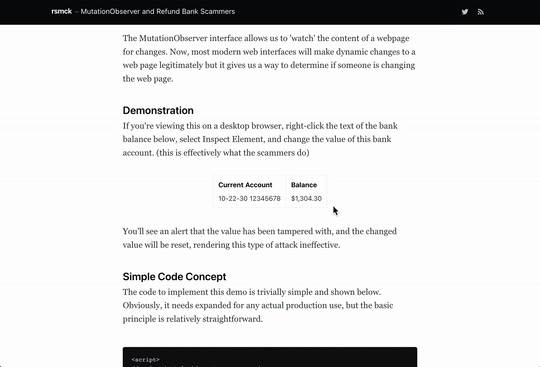
Simple Code Concept
The code to implement this demo is trivially simple and shown below. Obviously, it needs expanded for any actual production use, but the basic principle is relatively straightforward.
<script>
// we're just looking at one <span> here
// a real implementation could (and should) look at the whole page
var demoBalance = document.querySelector('span#bankBalanceDemo');
var obs = new MutationObserver(function(mutations) {
// perform some actions here
window.alert("Warning! Attempted unlawful interference");
});
// observe for changes
obs.observe(demoBalance, {
subtree: true,
characterData: true,
characterDataOldValue: true
});
</script>
Possible Actions
In the demo above, this renders a simple alert, but the point is simply detecting the actions. If I was a bank, I'd consider a more comprehensive and proactive response including possibly some (or all!) of the above actions;
- Display an error message. It's worth noting that the scammer will just close it if it appears immediately, and then spend some time trying to disable the code that generated it – a better action would be to 'queue' the message for a minute or so, such that the scammer believes their action is successful, then they'll continue to talk to the victim and the message will be displayed whilst the victim is reading the screen.
- End the session. This is a given, once such tampering takes place no other actions (even authenticated by two-factor authentication) should be valid on that session, completely preventing the scammer from transferring any money from the victim's account or the victim doing so under their instruction.
- Text or call the customer. The bank has alternative communication channels. We know the scammer is in control of their computer and might hide any messages that appear, this is an ideal opportunity to talk to the customer and ensure they don't fall victim to the scam. If a potential victim receives a text whilst on the phone to the scammer along the lines of "We've noticed unusual activity on your online banking, if you're on the phone to someone just now they cannot be trusted and you should hang up now and call your bank using the number on the back of your card"
Importantly, this would provide vital intelligence to the bank that their customer is - or could be - a potential victim of a scam at that exact moment.
Is this / could this be a product?
I discussed this idea with a few people and the consensus was 'you could sell that!' (and the thought of earning something from an idea is always appealing)
However, leaving aside my personal views on software patents, I probably could - but the market that would benefit most from such a thing is the banking sector and they've got their own developers and in-house code and are unlikely to use third party external services anyway, indeed I wouldn't want them to use a third party service on their online banking pages!
Above all this is a technique more than a product, one that isn't even particularly difficult, but one I'm surprised isn't more widely implemented!